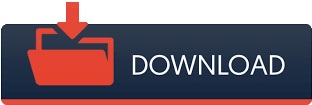
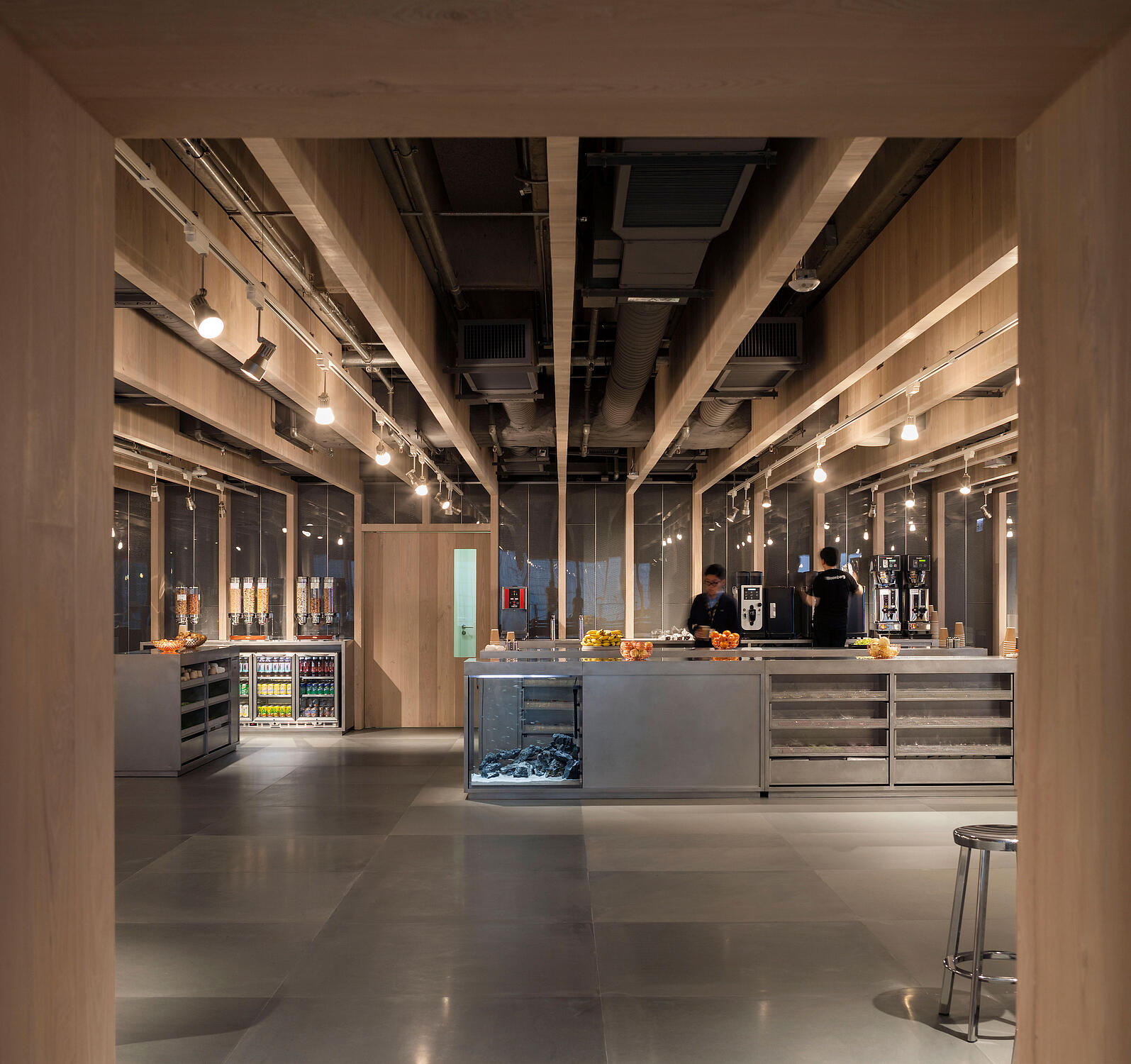
To make this possible we'll use a switch function to check which child object index is actually running the code and change what is drawn accordingly. The simplest thing to add here would be a draw_sprite() function with the light sprite, but in the demo, each of the child objects has a different origin offset, and it may be that you'd like to add in extra effects on a per-object basis. Time to add some lights!Īs mentioned above, the code/actions shown expect us to do something with the oLightParent object in the Draw Event code. If you run the project now, you should see the game as before, but now shrouded in darkness. You should go into the room editor now, and on the "Lighting" layer that you created previously, add the controller object we've just made (it will be automatically inherited by the other room, so no need to add it there). This helps keep memory use down and makes it less likely that your game will have issues running on low end hardware. We could make the surface the size of the room (and if you are not using cameras then that is what you would have to do), but in the demo the camera follows the player and so there is no need to draw anything that isn't going to be seen by the player. Finally the code is drawing the surface to the screen at the camera position. At this point we would draw the lights using the with statement, but we'll add that in a moment.

It then draws a rectangle to the surface with an 80% alpha (this is the "darkness" that we are going to illuminate), before changing to a subtractive blend mode. What this does is get the view camera position and size and stores these values in variables. Var _cy = camera_get_view_y(view_camera) Var _cx = camera_get_view_x(view_camera) We've got our if to check if the surface exists, so now we need to add an else for when it does exist, like this: else Why do we clear it? Well, a surface is really a memory location that has been "reserved" for drawing, and so if we don't clear the surface on creation, it may contain "garbage" from what was previously in the memory location and when we draw the surface we'll have unwanted artefacts. Var _ch = camera_get_view_height(view_camera) Īll we're doing here is checking to see if the surface exists and if it doesn't we create it and clear it. Var _cw = camera_get_view_width(view_camera) To catch this we'll be doing all our surface manipulation and drawing in the Draw Event.Īdd a Draw Event now to our oLightingcontroller and in it add the following: if !surface_exists(surf) We don't create it in this event however, as surfaces are volatile which means that they may be overwritten and removed from memory due to changes in the rendering (things like going fullscreen, or minimising the game will cause the surface to disappear, for example). Our lighting is going to use a surface with a subtractive blend mode - essentially we'll be drawing a black rectangle over the screen and then "subtracting" from it using a white sprite - and so we need a variable to hold the unique ID value of the surface we'll be using. In this event we'll add this simple piece of code: surf = -1 So, make a new object and call it " oLighting", then give it a Create Event. We need to make another object now to act as the controller for all the games lighting. With that done, we are now ready to start adding in our code to draw the lighting! This layer will be for our lighting controller object, so go ahead and name the layer " Lighting" now (note that there is no need to repeat this for the room rSand as it will inherit the layer automatically): To start with, you need to open up the room editor for the room rGrass, and then add a new instance layer. Once you have it imported the project into GMS2, run it and make sure it works and that you have an idea of how it is all put together.
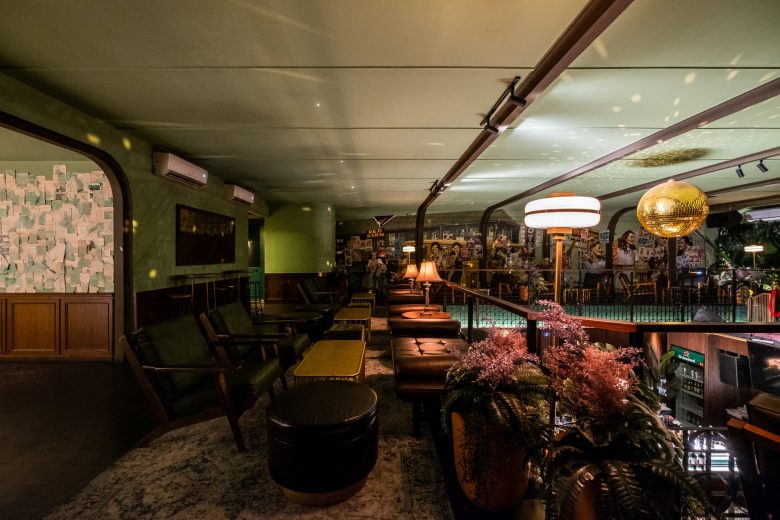
If you prefer to use DnD™ to make your games, we have a companion article for you here. NOTE: This tutorial is for people that use GML. Also for the sake of simplicity, we'll be basing this tutorial on the YoYo Platformer Demo, which you can get from the following link: By simple, we mean that these lights won't be able to cast shadows, but they will provide the cover of darkness along with areas of brightness, and can give a nice effect and are easy to modify so that they flicker (for example). Today's coffee-break tutorial is going to be about adding some very simple lighting effects to your games using surfaces.
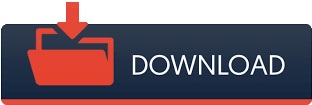